Have you ever wondered how to make Godot asynchronously load to Android View in multi-threads? Well, you’re in luck because we’ve got you covered! In Android development, it is possible to achieve this by using a few simple steps and some example code.
One way to accomplish this is by creating a new thread in your Android application and loading Godot asynchronously within that thread. This will allow Godot to run in the background while your main application continues to function without any interruptions.
You may also like to watch : Who Is Kamala Harris? Biography - Parents - Husband - Sister - Career - Indian - Jamaican Heritage
Here’s an example code snippet that demonstrates how to achieve this:
Thread godotThread = new Thread(new Runnable() {<br />
@Override<br />
public void run() {<br />
// Load Godot asynchronously here<br />
}<br />
});<br />
godotThread.start();<br />
```<br />
<br />
In the code above, we create a new thread called `godotThread` and implement the `run` method to load Godot asynchronously within that thread. By calling `godotThread.start()`, we kick off the thread and allow Godot to load in the background.<br />
<br />
By following this approach, you can ensure that Godot is loaded asynchronously to Android View in multi-threads, providing a seamless user experience without any performance issues. So go ahead and give it a try in your Android development projects! Thank us later!
How to Make Godot Asynchronously Loaded to Android View in Multi-Threads?
Are you looking to optimize your Android development process by asynchronously loading Godot to Android View in multi-threads? If so, you’ve come to the right place. In this article, we will provide you with a step-by-step guide on how to achieve this and even give you an example code to help you better understand the process.
What is Asynchronous Loading in Android Development?
Before we dive into the specifics of loading Godot asynchronously to Android View in multi-threads, let’s first understand what asynchronous loading means in the context of Android development. Asynchronous loading allows you to perform tasks in the background without blocking the main thread, thus providing a smoother user experience. This is especially important when dealing with resource-intensive tasks such as loading game engines like Godot.
Why Use Multi-Threads for Loading Godot in Android?
Using multi-threads for loading Godot in Android has several benefits. By utilizing multiple threads, you can distribute the workload across different cores of the device’s processor, thereby improving performance and responsiveness. Additionally, multi-threading allows you to execute tasks concurrently, enabling you to load Godot and other resources simultaneously without causing lag or delays.
You may also like to watch: Is US-NATO Prepared For A Potential Nuclear War With Russia - China And North Korea?
Step-by-Step Guide to Asynchronously Load Godot in Android View
Now that we have a better understanding of asynchronous loading and multi-threading, let’s walk through the steps to asynchronously load Godot to Android View in multi-threads.
1. **Create a Custom View with Godot Engine**: To begin, you’ll need to create a custom view that integrates the Godot engine into your Android application. This custom view will serve as the canvas where you will load and interact with your Godot game.
2. **Implement Asynchronous Loading**: Next, you’ll need to implement asynchronous loading logic in your custom view. This involves creating a separate thread or using a thread pool to load Godot and any associated resources in the background.
3. **Handle Resource Loading**: As Godot is a game engine, it requires various resources such as textures, scripts, and scenes to function properly. Make sure to handle the loading of these resources asynchronously to prevent any hiccups or delays during gameplay.
4. **Update UI on Main Thread**: While loading Godot asynchronously in multi-threads, it’s crucial to update the UI on the main thread. This ensures that any changes or interactions with the game are reflected in real-time, providing a seamless user experience.
5. **Example Code for Asynchronously Loading Godot in Android View**:
“`java
// Create a custom view with Godot Engine
public class GodotView extends View {
public GodotView(Context context) {
super(context);
// Initialize Godot Engine
GodotLib.initialize(context);
}
// Implement asynchronous loading logic
public void loadGodotAsync() {
new Thread(new Runnable() {
@Override
public void run() {
// Load Godot resources asynchronously
GodotLib.loadResources();
}
}).start();
}
// Handle resource loading
public void onResourceLoaded() {
// Handle resource loading logic here
}
// Update UI on the main thread
public void updateUI() {
post(new Runnable() {
@Override
public void run() {
// Update UI elements here
}
});
}
}
“`
By following these steps and utilizing the example code provided, you can effectively load Godot asynchronously to Android View in multi-threads, enhancing the performance and user experience of your Android application.
In conclusion, asynchronous loading and multi-threading are essential techniques in Android development, especially when working with resource-intensive tasks like loading game engines. By following the steps outlined in this article and utilizing the example code provided, you can successfully integrate Godot into your Android application and provide a seamless user experience for your players.
If you’re interested in learning more about Android development and game engines like Godot, be sure to check out the following sources for further reading:
– [Android Developer Guide](https://developer.android.com/guide)
– [Godot Engine Documentation](https://docs.godotengine.org/en/stable/)
– [Multi-Threading in Android](https://developer.android.com/guide/components/processes-and-threads)
Remember, practice makes perfect, so don’t hesitate to experiment with different techniques and approaches to find what works best for your specific project. Happy coding!
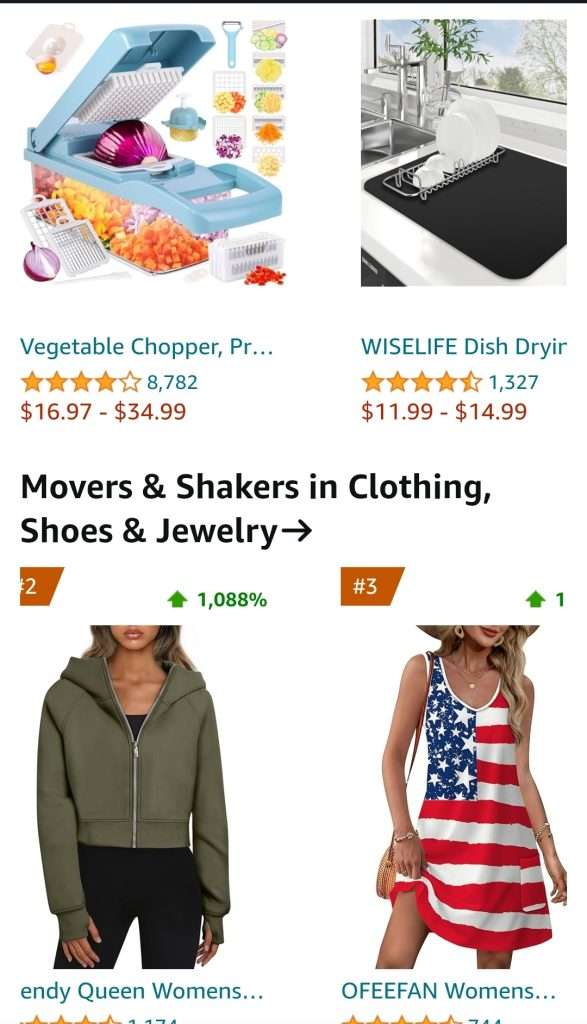
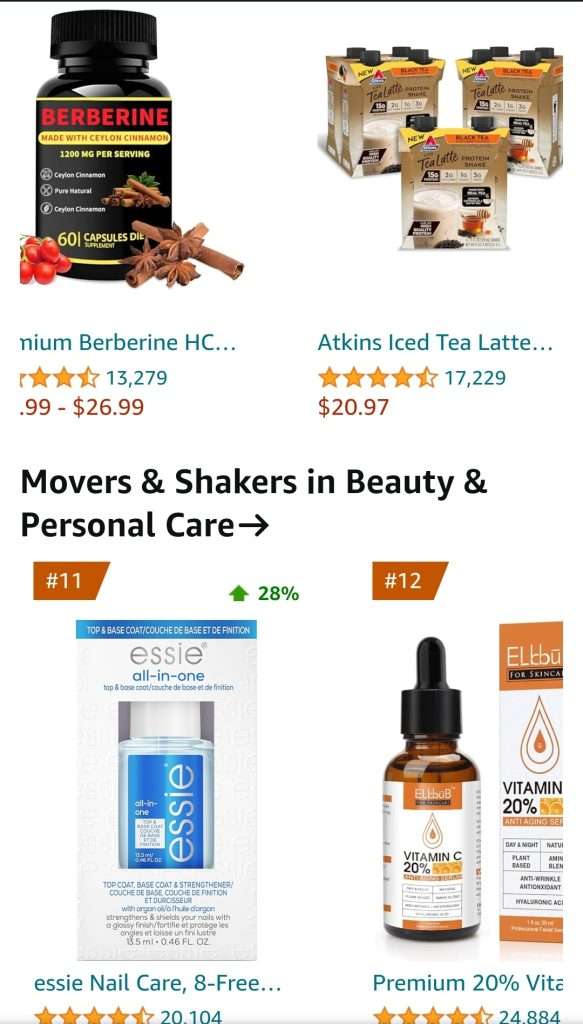
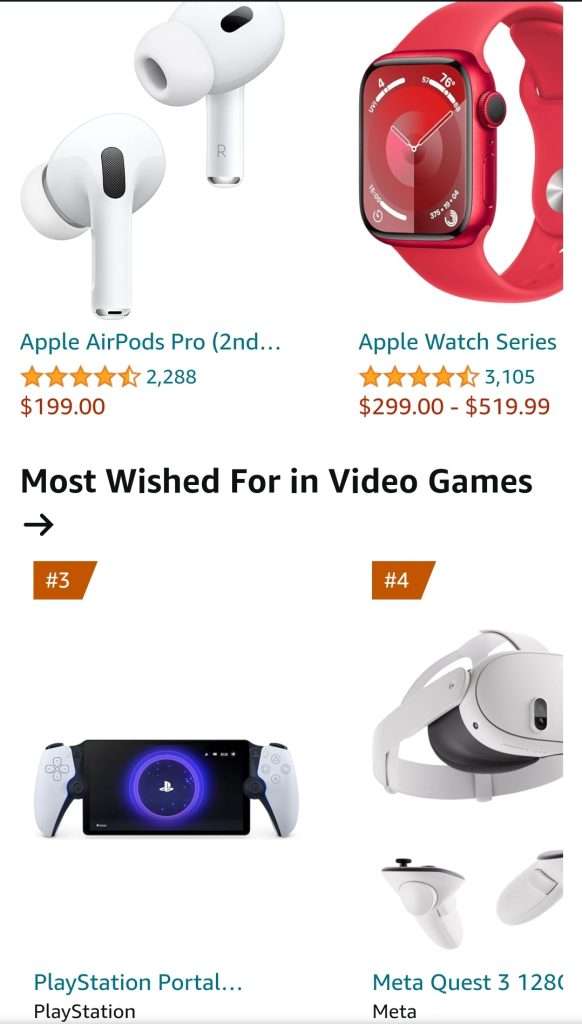
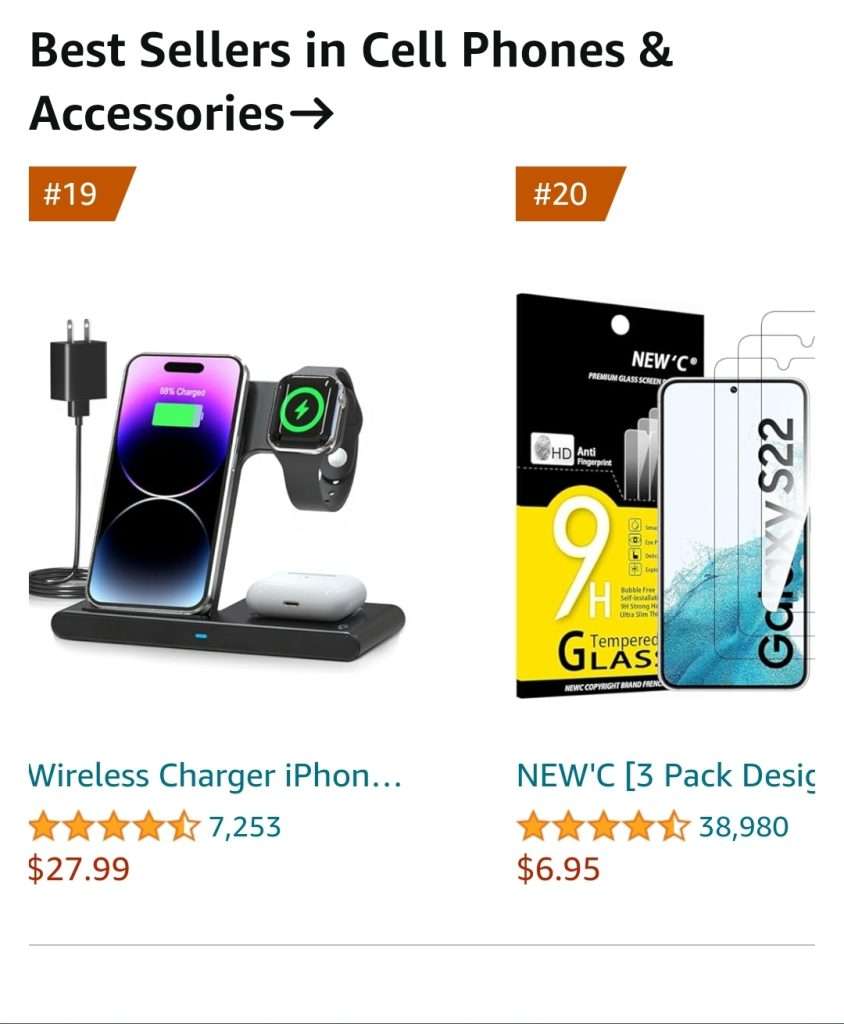