Are you looking to build powerful and scalable RESTful APIs using Golang? Look no further than this comprehensive guide on building RESTful web services with Go. Whether you’re a beginner or an experienced developer, this resource will help you master the art of creating robust APIs that can handle heavy loads with ease.
With step-by-step instructions and practical examples, you’ll learn everything you need to know to build RESTful web services that scale gracefully. From setting up your development environment to designing API endpoints and handling authentication, this guide covers it all.
You may also like to watch : Who Is Kamala Harris? Biography - Parents - Husband - Sister - Career - Indian - Jamaican Heritage
By the end of this tutorial, you’ll have the knowledge and skills to create high-performance RESTful APIs that meet the demands of modern web applications. So why wait? Dive into the world of building RESTful web services with Go today and take your programming skills to the next level. Happy coding!
If you are looking to build powerful RESTful Web services with Go, you have come to the right place. In this article, we will guide you through the process of creating RESTful APIs using the Go programming language. Whether you are a beginner or an experienced developer, this step-by-step guide will help you scale your APIs gracefully and efficiently. So, let’s dive in and explore how you can leverage the power of Go to build robust web services.
What is Go Programming Language?
Before we delve into building RESTful Web services with Go, let’s first understand what the Go programming language is all about. Developed by Google in 2007, Go, also known as Golang, is an open-source programming language designed for building simple, reliable, and efficient software. With its strong focus on simplicity and performance, Go has gained popularity among developers for its ease of use and speed.
Why Use Go for Building RESTful Web Services?
There are several reasons why Go is an excellent choice for building RESTful Web services. One of the key advantages of using Go is its concurrency support, which allows you to write highly efficient and scalable code. Additionally, Go’s static typing system helps catch errors at compile time, making your code more robust and reliable. Moreover, Go’s standard library includes built-in support for HTTP servers and clients, making it easy to create RESTful APIs without relying on third-party libraries.
You may also like to watch: Is US-NATO Prepared For A Potential Nuclear War With Russia - China And North Korea?
Setting Up Your Development Environment
Before you start building RESTful Web services with Go, you need to set up your development environment. To do this, you will first need to install Go on your machine. You can download the latest version of Go from the official website (https://golang.org/). Once you have installed Go, you can create a new project directory and start writing your Go code.
Creating a Simple HTTP Server
To create a simple HTTP server in Go, you can use the built-in `net/http` package. This package provides functions for handling HTTP requests and responses, making it easy to build RESTful APIs. Here is an example of a simple HTTP server that listens on port 8080 and responds with “Hello, World!” to incoming requests:
“`go
package main
import (
“fmt”
“net/http”
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, “Hello, World!”)
}
func main() {
http.HandleFunc(“/”, handler)
http.ListenAndServe(“:8080”, nil)
}
“`
In this example, we define a `handler` function that writes “Hello, World!” to the response writer `w`. We then register this handler with the `/` route using `http.HandleFunc`, and start the HTTP server on port 8080 with `http.ListenAndServe`.
Implementing RESTful Endpoints
Now that you have set up a basic HTTP server, it’s time to implement RESTful endpoints for your API. RESTful APIs typically use standard HTTP methods such as GET, POST, PUT, and DELETE to perform CRUD (Create, Read, Update, Delete) operations on resources. Here is an example of how you can create RESTful endpoints for a simple todos API:
“`go
package main
import (
“encoding/json”
“fmt”
“net/http”
)
type Todo struct {
ID int `json:”id”`
Title string `json:”title”`
Done bool `json:”done”`
}
var todos []Todo
func getTodos(w http.ResponseWriter, r *http.Request) {
json.NewEncoder(w).Encode(todos)
}
func addTodo(w http.ResponseWriter, r *http.Request) {
var newTodo Todo
json.NewDecoder(r.Body).Decode(&newTodo)
todos = append(todos, newTodo)
json.NewEncoder(w).Encode(newTodo)
}
func main() {
http.HandleFunc(“/todos”, getTodos)
http.HandleFunc(“/todos/add”, addTodo)
http.ListenAndServe(“:8080”, nil)
}
“`
In this example, we define a `Todo` struct to represent todo items with an `ID`, `Title`, and `Done` field. We then create two HTTP handlers `getTodos` and `addTodo` to retrieve and add todos, respectively. Finally, we register these handlers with the `/todos` and `/todos/add` routes and start the HTTP server on port 8080.
Conclusion
In this article, we have explored how you can build RESTful Web services with Go. By leveraging Go’s concurrency support, static typing system, and built-in HTTP package, you can create efficient and scalable APIs that meet your business needs. Whether you are building a simple todos API or a complex microservices architecture, Go provides the tools and capabilities to help you succeed. So, start coding with Go today and unlock the full potential of building RESTful Web services.
Building RESTful Web services with Go: Learn how to build powerful RESTful APIs with Golang that scale gracefully https://t.co/NQSx5jzQYs
— PCPages (@CERBAFASHION) July 28, 2024
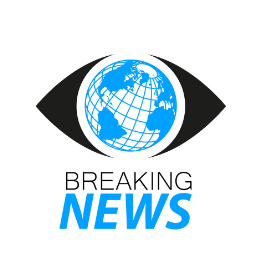
Building RESTful Web services with Go: Learn how to build powerful RESTful APIs with Golang that scale gracefully
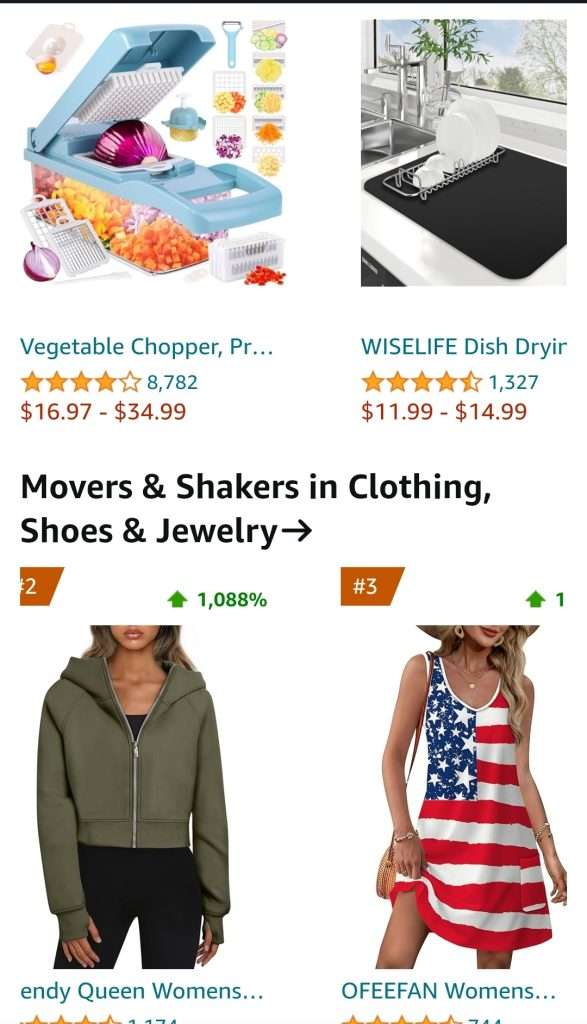
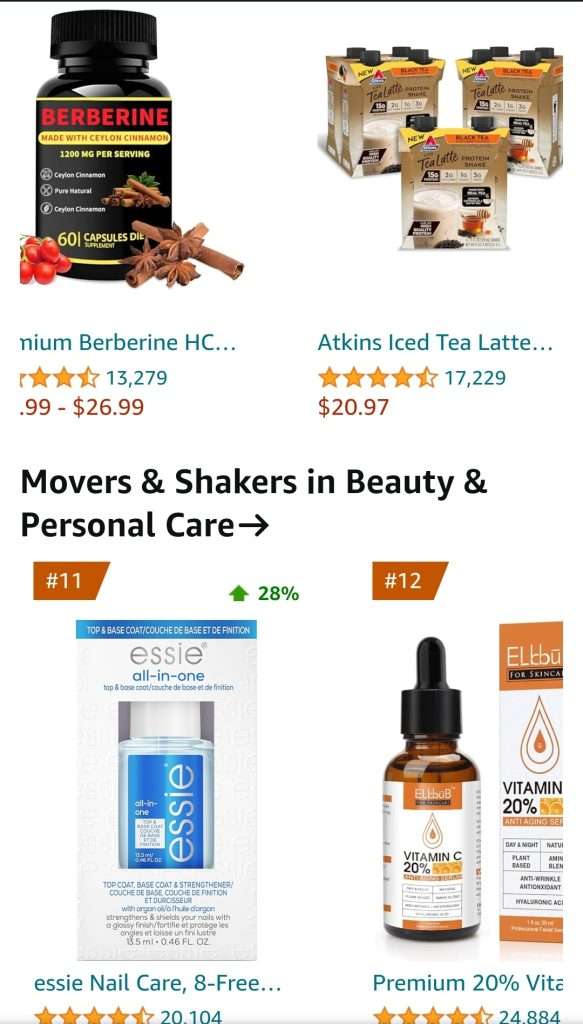
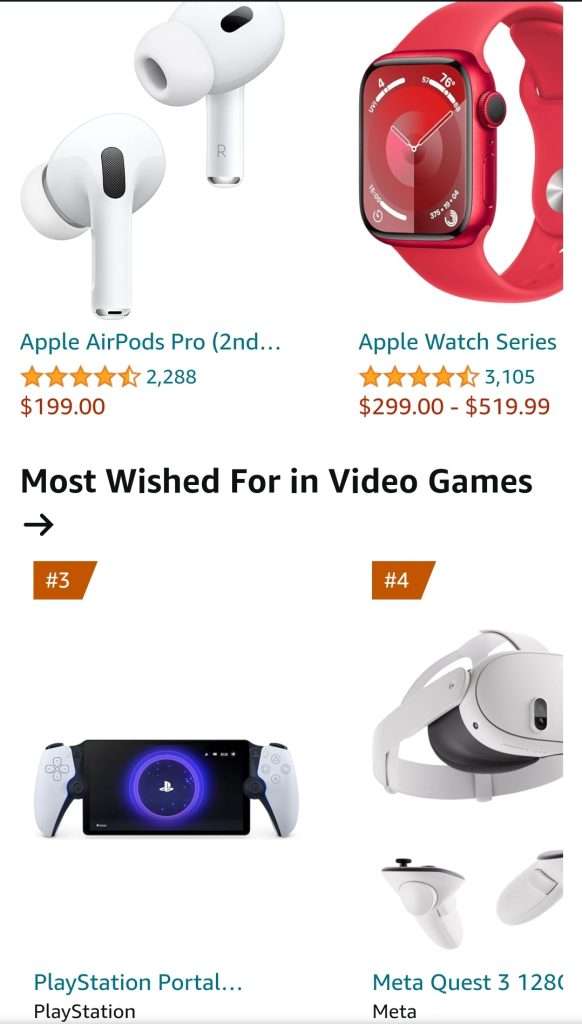
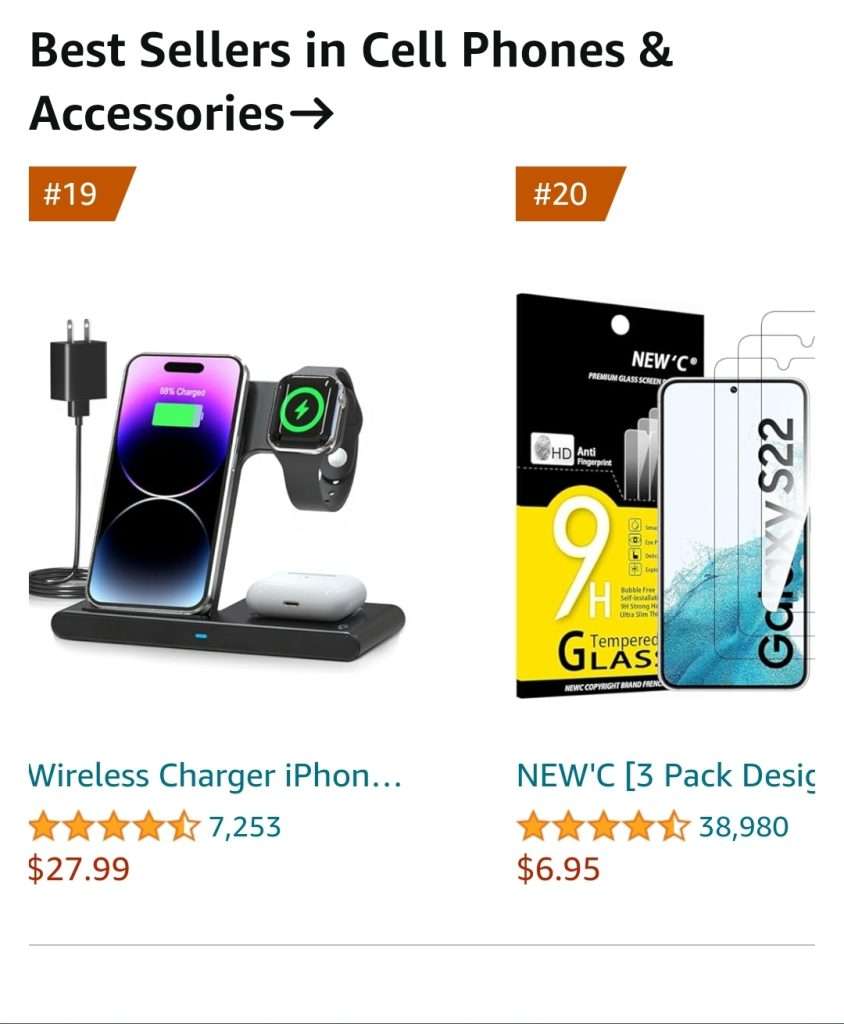