Calculate Average Word Length in Python: A Step-by-Step Guide
If you’re looking to calculate the average word length in Python, you’re in the right place! In this tutorial, we will walk you through the process of writing a Python script that will take a text input and output the average length of the words in the text.
You may also like to watch : Who Is Kamala Harris? Biography - Parents - Husband - Sister - Career - Indian - Jamaican Heritage
To get started, you’ll need to have Python installed on your computer. If you don’t already have it, you can easily download and install it from the official Python website. Once you have Python up and running, you can follow along with the steps outlined in this tutorial.
First, you’ll need to define a function that will calculate the average word length. You can do this by splitting the input text into individual words and then calculating the length of each word. Next, you’ll sum up the lengths of all the words and divide by the total number of words to get the average word length.
Here’s a sample Python script that accomplishes this task:
“`python
def average_word_length(text):
words = text.split()
total_length = sum(len(word) for word in words)
return total_length / len(words)
You may also like to watch: Is US-NATO Prepared For A Potential Nuclear War With Russia - China And North Korea?
text = “This is a sample text to calculate average word length”
avg_length = average_word_length(text)
print(f”The average word length is: {avg_length}”)
“`
In this script, we first define the `average_word_length` function, which takes a text input as a parameter. We then split the text into individual words using the `split` method and calculate the total length of all the words using a generator expression. Finally, we return the average word length by dividing the total length by the number of words.
You can test this script by providing your own text input and running the script. It will output the average word length of the text you provided.
Calculating the average word length in Python can be a useful tool for analyzing text data. You can use this script to gain insights into the complexity of a piece of text or to compare the average word lengths of different texts.
In conclusion, this tutorial has shown you how to calculate the average word length in Python using a simple script. By following the steps outlined in this tutorial, you can easily write your own Python script to calculate the average word length of any text input. So give it a try and see what insights you can uncover from your text data!
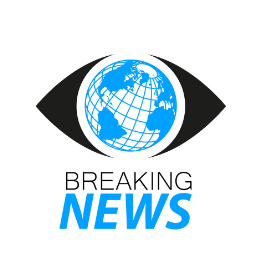
Calculate Average Word Length in Python!
**Who is Python?**
Python is a high-level, interpreted programming language that is widely used in various fields such as web development, data science, artificial intelligence, and more. It was created by Guido van Rossum and first released in 1991. Python is known for its simplicity and readability, making it a popular choice among developers of all levels.
**What is Average Word Length?**
Average word length is a metric used to determine the average number of characters in a word. This can be useful in various applications, such as text analysis, natural language processing, and more. By calculating the average word length, we can gain insights into the structure and complexity of a piece of text.
**How to Calculate Average Word Length in Python?**
Now, let’s dive into the step-by-step process of calculating the average word length in Python. We will be using a simple script to achieve this task.
1. **Input the Text**: The first step is to input the text for which you want to calculate the average word length. You can either hardcode the text into the script or prompt the user to input the text.
2. **Tokenize the Text**: Next, we need to tokenize the text into words. This can be done using the `split()` method in Python, which splits the text into a list of words based on whitespace.
3. **Calculate Word Length**: Once we have tokenized the text, we can loop through each word in the list and calculate its length using the `len()` function in Python.
4. **Calculate Average**: Finally, we can calculate the average word length by summing up the lengths of all words and dividing by the total number of words.
**Example Script to Calculate Average Word Length in Python:**
Here is a simple Python script that calculates the average word length in a given text:
“`python
text = “Lorem ipsum dolor sit amet, consectetur adipiscing elit”
words = text.split()
total_length = sum(len(word) for word in words)
average_length = total_length / len(words)
print(“Average Word Length:”, average_length)
“`
By running this script, you will be able to calculate the average word length in the provided text.
**Why is Average Word Length Important?**
Average word length can provide valuable insights into the complexity and readability of a piece of text. In fields such as linguistics, literature analysis, and natural language processing, average word length is often used as a key metric to analyze and compare texts.
In conclusion, calculating the average word length in Python is a simple yet powerful task that can provide valuable insights into the structure and complexity of a piece of text. By following the steps outlined in this article, you will be able to easily calculate the average word length in any given text source.
For more information on Python programming and text analysis, check out this [Python programming guide](https://www.python.org/).
Remember, practice makes perfect, so don’t hesitate to try out different texts and experiment with the script to further enhance your understanding of average word length calculation in Python. Happy coding!
https://www.youtube.com/watch?v=96t_tmBwHww
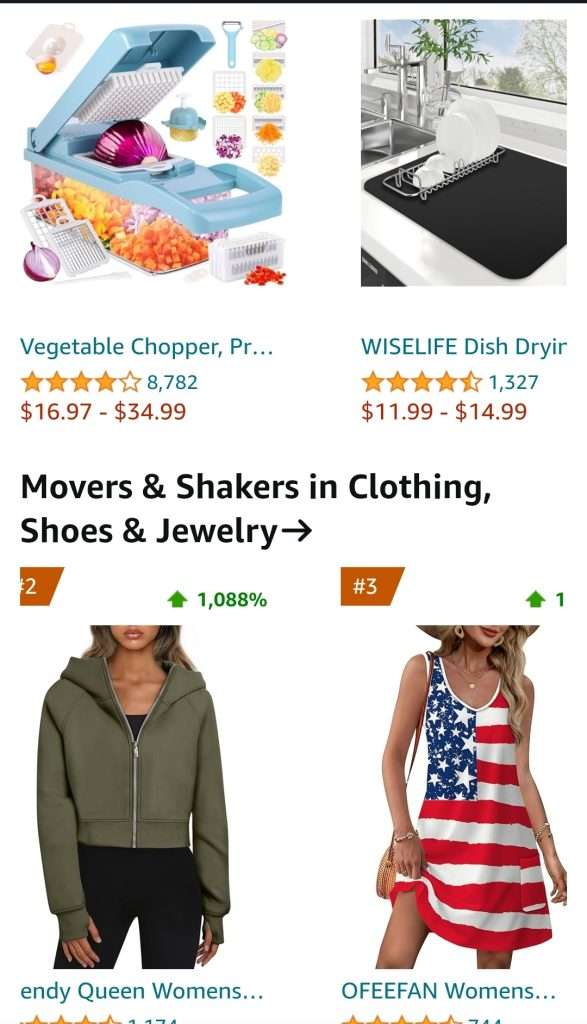
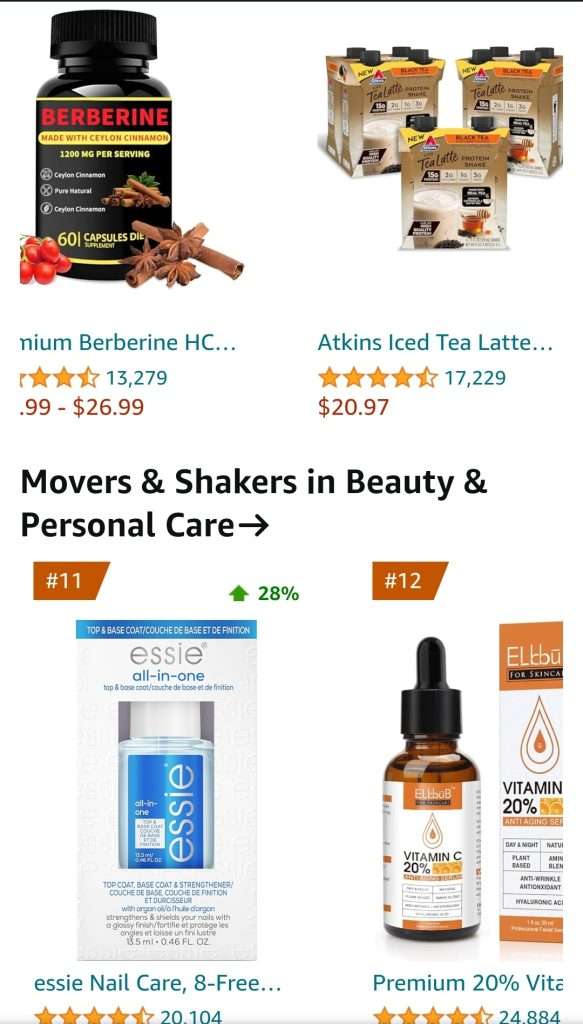
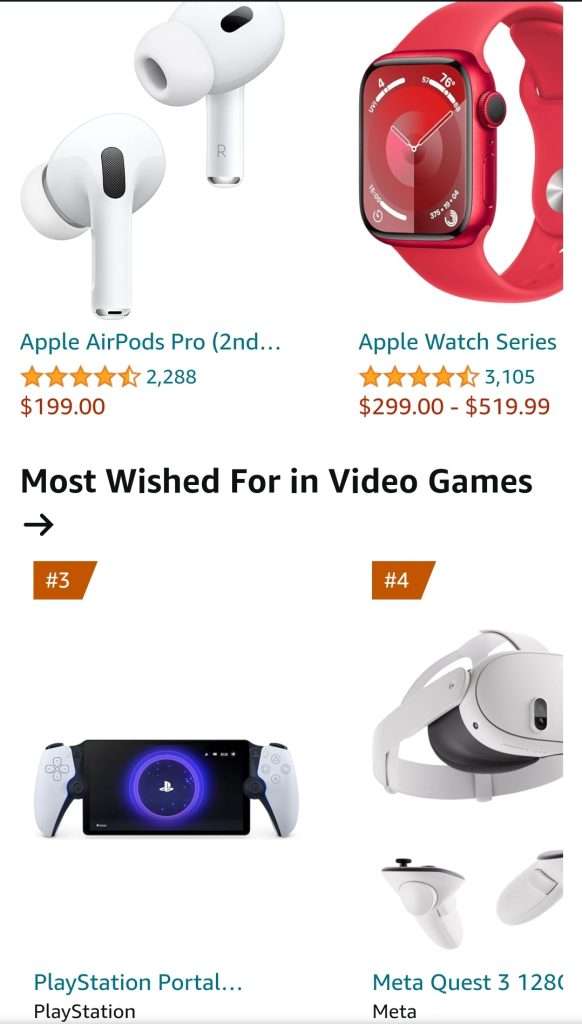
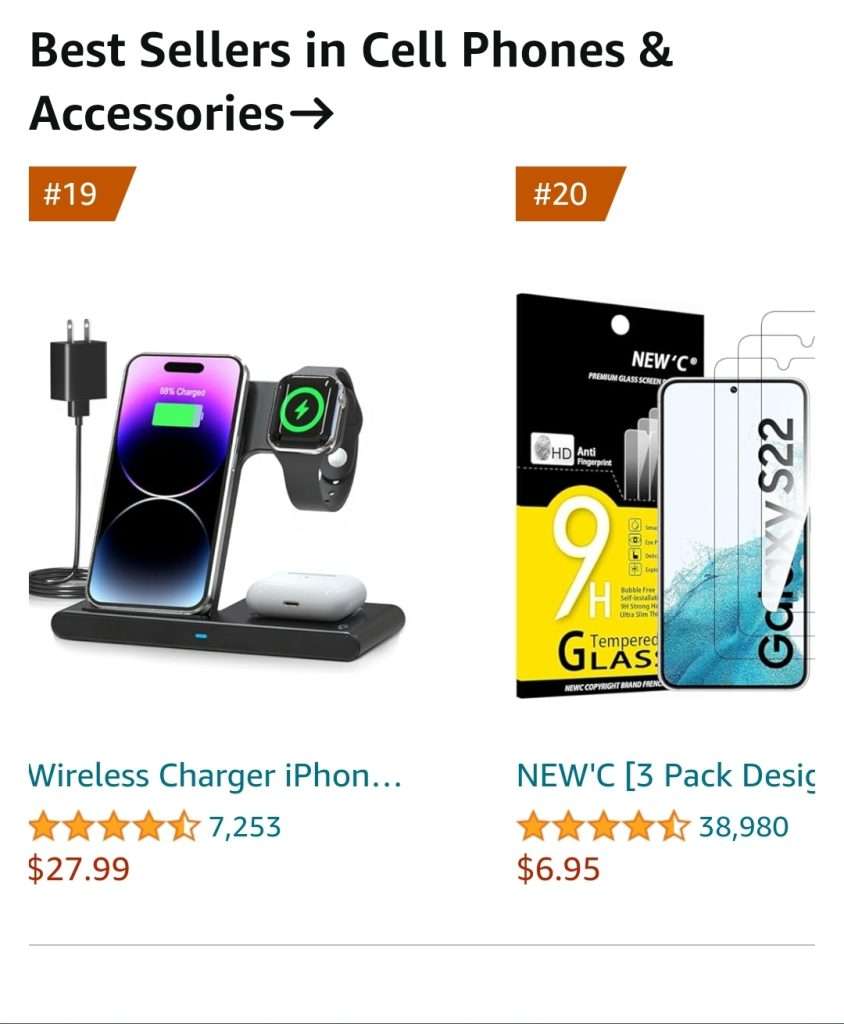