Step by Step Guide to Solving Diameter of Binary Tree LeetCode Solution
Are you struggling with the Diameter of Binary Tree LeetCode problem? Don’t worry, we’ve got you covered! In this step-by-step guide, we will walk you through the solution to this challenging problem. By the end of this guide, you will have a clear understanding of how to solve the Diameter of Binary Tree problem efficiently.
You may also like to watch : Who Is Kamala Harris? Biography - Parents - Husband - Sister - Career - Indian - Jamaican Heritage
To begin solving this problem, first, let’s understand what the Diameter of a Binary Tree is. The diameter of a binary tree is the number of nodes on the longest path between two end nodes in the tree. In other words, it is the length of the longest path between any two nodes in a tree.
One approach to solving this problem is by using recursion. We can calculate the diameter of a binary tree by recursively calculating the height of the left and right subtrees of each node and then finding the maximum diameter.
The first step in solving this problem is to define a recursive function that calculates the height of a binary tree. This function will take a node as input and return the height of the subtree rooted at that node. We can define this function as follows:
“`python
def height(node):
if not node:
return 0
left_height = height(node.left)
right_height = height(node.right)
return 1 + max(left_height, right_height)
“`
You may also like to watch: Is US-NATO Prepared For A Potential Nuclear War With Russia - China And North Korea?
Next, we can define another recursive function that calculates the diameter of a binary tree. This function will take a node as input and return the diameter of the subtree rooted at that node. We can define this function as follows:
“`python
def diameterOfBinaryTree(node):
if not node:
return 0
left_height = height(node.left)
right_height = height(node.right)
left_diameter = diameterOfBinaryTree(node.left)
right_diameter = diameterOfBinaryTree(node.right)
return max(left_height + right_height, max(left_diameter, right_diameter))
“`
By using these two recursive functions, we can efficiently calculate the diameter of a binary tree. This approach allows us to break down the problem into simpler subproblems and solve them recursively.
In conclusion, the Diameter of Binary Tree LeetCode problem can be solved efficiently using a recursive approach. By calculating the height of each subtree and finding the maximum diameter, we can determine the diameter of the binary tree. By following the step-by-step guide outlined above, you can confidently tackle this problem and improve your problem-solving skills. So, what are you waiting for? Give it a try and see the results for yourself!
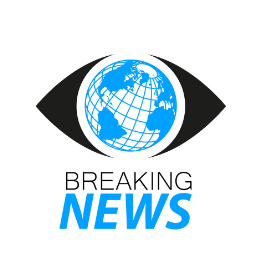
Diameter of Binary Tree LeetCode Solution Step by Step Guide to Solving the Problem
### What is the Diameter of Binary Tree LeetCode Problem?
The Diameter of Binary Tree problem on LeetCode asks you to find the length of the longest path between any two nodes in a binary tree. This path may or may not pass through the root of the tree. The length of a path is defined as the number of edges between the nodes.
### How to Approach the Diameter of Binary Tree Problem?
To solve the Diameter of Binary Tree problem, we need to calculate the maximum depth of the left and right subtrees for each node in the binary tree. We can then find the sum of the maximum depths of the left and right subtrees for each node and update the diameter of the tree accordingly.
### Step 1: Define the TreeNode Class
The first step in solving the Diameter of Binary Tree problem is to define the TreeNode class that represents a node in the binary tree. This class should have attributes for the value of the node, as well as references to its left and right children.
“`java
class TreeNode {
int val;
TreeNode left;
TreeNode right;
public TreeNode(int val) {
this.val = val;
}
}
“`
### Step 2: Implement the Recursive Function
Next, we need to implement a recursive function that calculates the maximum depth of a subtree rooted at a given node. This function will return the maximum depth of the subtree and update the diameter of the tree if necessary.
“`java
public int maxDepth(TreeNode node) {
if (node == null) {
return 0;
}
int leftDepth = maxDepth(node.left);
int rightDepth = maxDepth(node.right);
diameter = Math.max(diameter, leftDepth + rightDepth);
return 1 + Math.max(leftDepth, rightDepth);
}
“`
### Step 3: Calculate the Diameter of the Binary Tree
Finally, we can calculate the diameter of the binary tree by calling the `maxDepth` function on the root node of the tree. This will update the `diameter` variable with the length of the longest path between any two nodes in the tree.
“`java
int diameter = 0;
public int diameterOfBinaryTree(TreeNode root) {
maxDepth(root);
return diameter;
}
“`
By following these steps and implementing the recursive function to calculate the maximum depth of the binary tree, you can successfully solve the Diameter of Binary Tree problem on LeetCode.
In conclusion, the Diameter of Binary Tree problem on LeetCode is a challenging algorithmic problem that requires a deep understanding of binary trees and recursive functions. By following the step-by-step guide provided in this article, you can improve your problem-solving skills and tackle similar challenges with confidence.
For more information on the Diameter of Binary Tree problem and other coding challenges, you can refer to the official LeetCode website [here](https://leetcode.com/problems/diameter-of-binary-tree/). Happy coding!
https://www.youtube.com/watch?v=a_JBDpikYKY
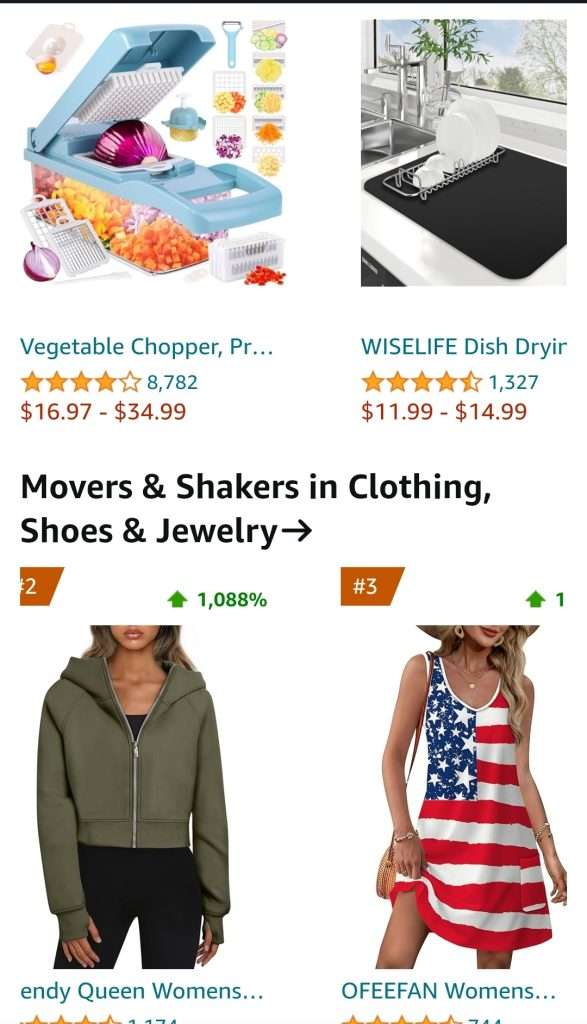
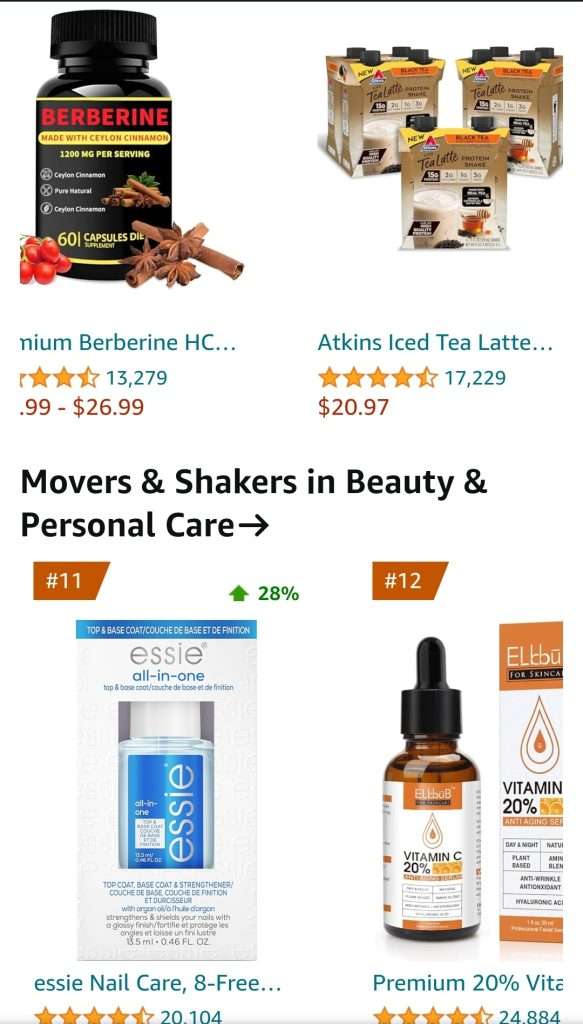
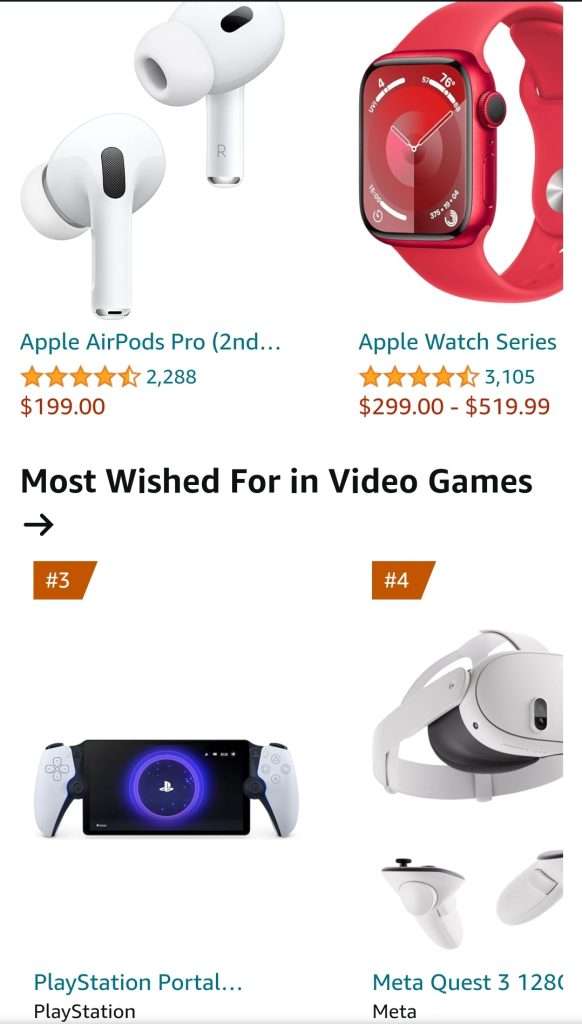
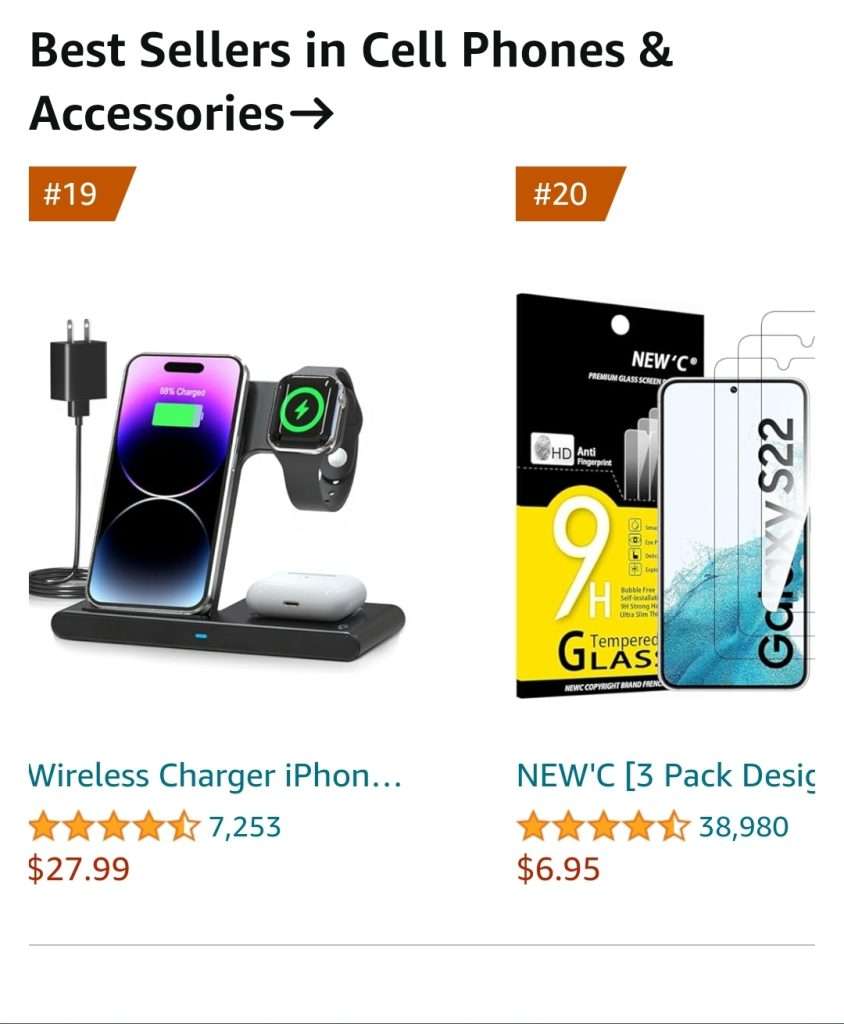